Level Up Your Knowledge About JavaScript Strings with This Article!
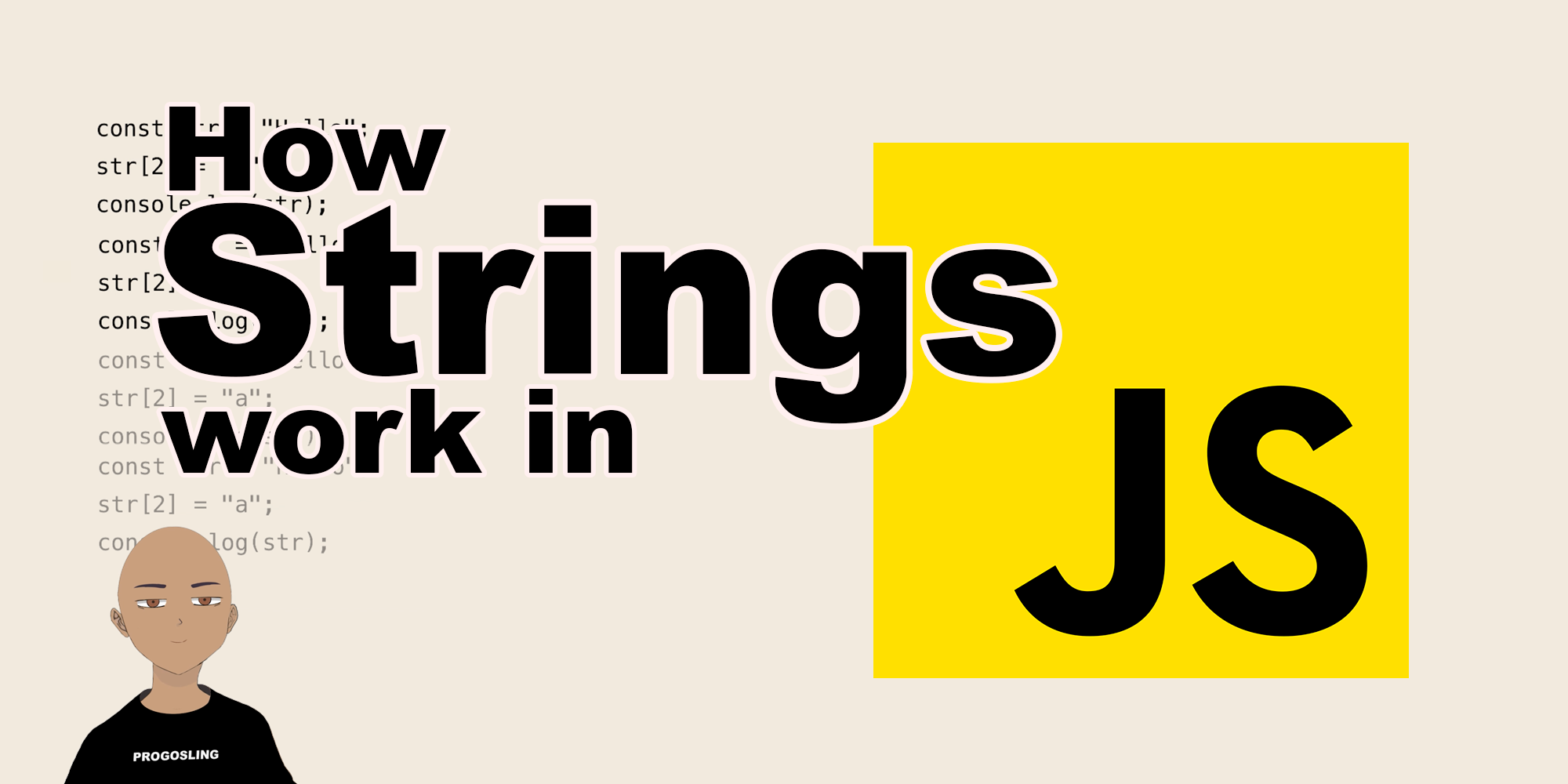
A short article about how strings work in JavaScript. It covers string immutability, comparison mechanisms, and memory allocation optimization for strings.
Let's start with some questions: what will this code output?
const str = "Hello";
str[2] = "a";
console.log(str);
And what if we replace const
with let
?
let str = "Hello";
str[2] = "a";
console.log(str);
Try entering this code into your browser console and see what happens.
In JavaScript, strings are immutable. This means that regardless of whether the variable is declared with let
or const
, the value will not change, and in both cases, "Hello"
will be output.
But what if we add an assignment operator with string concatenation:
const str = "Hello";
str += " World";
console.log(str);
This code will result in a TypeError: Assignment to constant variable
because we declared the string as const
. Let's change it to let
:
let str = "Hello";
str += " World";
console.log(str);
In JavaScript, strings are copied when assigned a value from one string to another. Therefore, this code will output "Hello World"
. At the end of the article, we will step by step explain how such code works.
Let's delve deeper into string copying. What will this code output and why?
const str1 = "Hello";
const str2 = str1;
console.log(str1 === str2);
When copying a string in JavaScript, a new reference to the string object is created, but the string itself is not duplicated in memory. Therefore, when comparing str1 === str2
, true
will be output. Here's a detailed breakdown:
- Creating the string
'Hello'
: Memory allocation for storing the string'Hello'
. - Assigning a value to another variable: Variable
str2
gets a reference to the same string object in memory as variablestr1
. - Variable comparison: When comparing
str1 === str2
, JavaScript first compares the reference values, not the strings themselves. Since both variables reference the same string object in memory, the comparison will returntrue
.
But what if we don't copy the string and instead create a new one with the same value as the first one? What will this code output, why, and how does it work?
const str1 = "Hello";
const str2 = "Hello";
console.log(str1 === str2);
- When
const str1 = 'Hello'
is declared, memory is allocated to store the string'Hello'
. - Then, when
const str2 = 'Hello'
is declared, JavaScript optimizes memory usage and creates only one string object for both variables. Instead of allocating new memory for the string'Hello'
, JavaScript detects that the string'Hello'
already exists in memory (as a result of declaringstr1
) and simply creates a reference to this same string for variablestr2
. - When
console.log(str1 === str2)
is executed, a comparison is made between variablesstr1
andstr2
. Since both variables reference the same string object in memory, the comparison will returntrue
.
Now, let's examine concatenation of strings in more detail:
const str1 = "Hello";
const str2 = str1 + "2";
console.log(str1 === str2);
- When
const str1 = 'Hello'
is declared, memory is allocated for the string'Hello'
. - When
const str2 = str1 + '2'
is declared, string concatenation occurs. JavaScript creates a new string by combining the contents ofstr1
(which contains'Hello'
) and the string'2'
. Since strings in JavaScript are immutable, a new string object is created for the concatenation result, in this case'Hello2'
. - When
console.log(str1 === str2)
is executed, sincestr1
contains'Hello'
andstr2
contains'Hello2'
, they point to different string objects in memory, and the comparison will returnfalse
.